Introduction
Built-In vs external package: Here are two essential methods of how you can find out if two Flutter objects are equal or not.
Equality comparison is essential in any kind of app. Duplicate objects are often undesired. In this article I’ll demonstrate how you can find out if two Flutter objects are equal or not. You might probably know the drill from other programming languages and Dart makes no exception here. The article will show you two ways of comparison. A longer one with built-in methods provided by Dart and another one that relies on an external package but is less verbose.
Compare with built-in methods
Let’s assume we have a simple class with an identifier, a name, and an email property. When using the options provided by the Dart language, you would override two methods:
- The equality operator
==
- And the method
hashCode
Here is a code example:
class Person {
final int id;
final String name;
final String email;
Person(this.id, this.name, this.email);
// Overriding the equality operator
@override
bool operator ==(Object other) {
if (identical(this, other)) return true;
if (other is! Person) return false;
return id == other.id &&
name == other.name &&
email == other.email;
}
// Overriding the hashCode method
@override
int get hashCode => id.hashCode ^ name.hashCode ^ email.hashCode;
}
Ok, let’s break down what happens in this code.
First, the operator ==
is overridden. The code uses the identical function to determine if the two objects share the same reference. If not, then a type check is performed. In case the types match, all property values are compared.
The second step is to override hashCode
. This ensures that objects with the same property values are considered equal because they return the same hash value. It’s also useful for efficient lookups in hash-based collections like HashMap or HashSet.
While this is straight forward, it can get quite verbose when you have more properties. The second approach solves this problem for you.
Compare with the equatable package
The package equatable will do everything for you that I described above and save you many lines of code. The only things you need to make your object derive from Equatable
and specify the properties you want to include in the comparison.
Here is an example:
import 'package:equatable/equatable.dart';
class Person extends Equatable {
final int id;
final String name;
final String email;
Person(this.id, this.name, this.email);
@override
List<Object?> get props => [id, name, email];
}
The props
getter returns all properties that should be taken into consideration when comparing objects.
Sometimes, your objects have unique identifiers from a database. In this case it would be enough to just compare the id
property and skip the others.
This approach requires way less lines of code to achieve the same result. But you have the downside of relying on (another) 3rd party package.
Bonus: Generate data classes quickly
Dart Quicktype is a great service that converts JSON into clean Dart objects. It has many configuration options to make your class exactly how you need it. There is also an equatable option which I highly recommend.
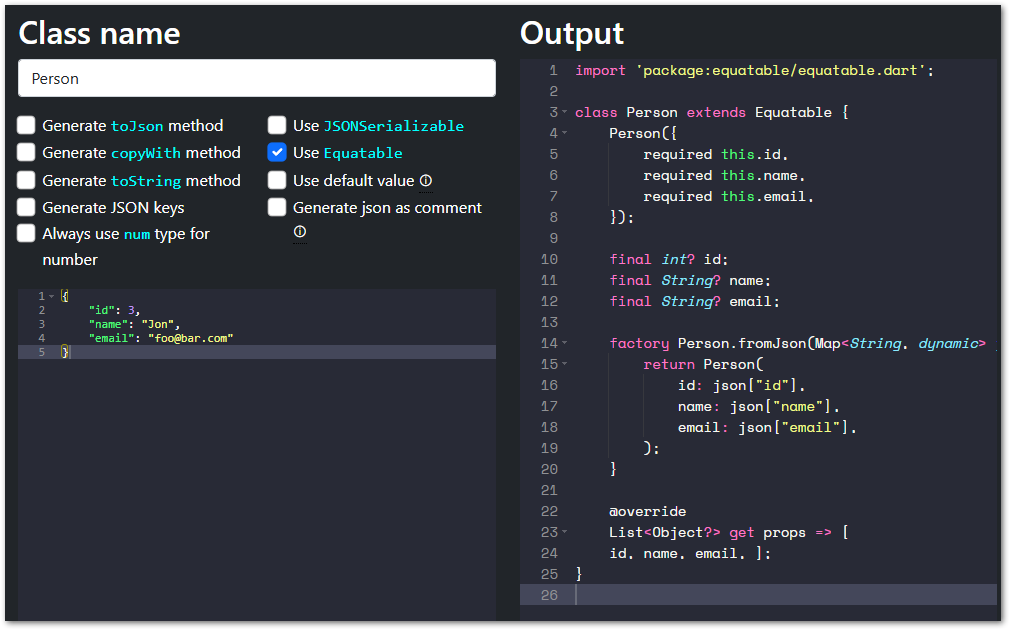
Of course, your favorite AI model can probably do the same for you but in my experience there is often manual editing required. I use this service instead whenever I can.
Conclusion
In this article I showed you two ways how you can find out if two Flutter objects are equal or not. You can do it yourself to not rely on another 3rd party package. Or you can just the equatable package and save many lines of code. Both ways will work fine.
Related articles
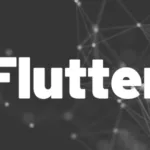
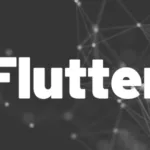