Introduction
Let’s have a look at how Widget testing in Flutter apps works. Improve your testing knowledge with this guide.
Flutter offers several types of tests that can be used to ensure correct app behavior. There are unit tests, integration tests, and finally widget tests. In this article, we’ll focus on widget tests. This means that a part of the app is rendered during the tests and you can describe test steps to copy the behavior of a real user. The Flutter SDK offers methods to simulate a complete workflow.
The app to test
I created a simple app that needs some widget tests. You can see a demo below.
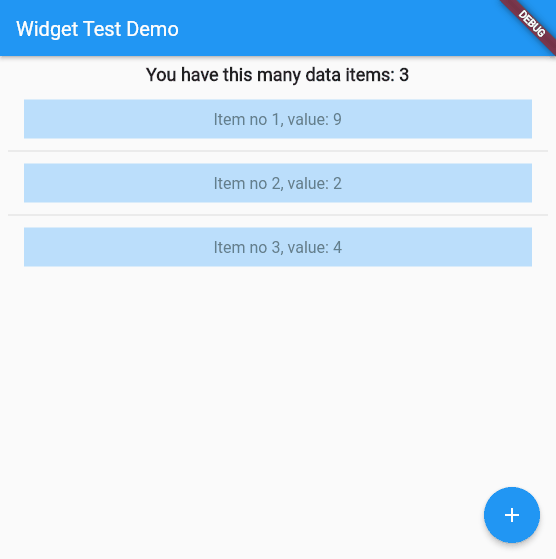
The app contains a Scaffold with a static AppBar, a description Text, a ListView, and a FloatingActionButton. When you click on the button, a new row is inserted into the ListView with a random number between 0 and 10, the header text is updated, and a SnackBar is shown. You can also delete entries with a long press. A simple tap shows a confirmation of what row you clicked. This is what we are going to test now. ?
Writing the tests
As the app workflow is pretty simple, we’ll write just one test that fulfills all requirements. You can, of course, split your tests into multiple ones if it makes sense. Good test organization is essential in larger apps, so take your time and think about what’s reasonable.
Our test will do the following: (? action step, ? verification step)
- Verify that there are already 3 data items present
- Tap the FloatingActionButton
- Verify that a new data item has been added
- Tap the 3rd item
- Verify that the SnackBar shows the same data as in the list
- Long press the 2nd item
- Verify that the correct item has been deleted
The test skeleton for widget tests is similar to unit tests but has an additional WidgetTester argument. It always looks like this
WidgetTester
The WidgetTester is the central unit that performs actions during the test. For example, there are methods to
▶ write into TextField widgets → enterText
▶ tap widgets → tap
▶ trigger animations and rendering → pump, pumpAndSettle
Finding widgets
To find widgets, you use the Finder class. It has many methods to find exactly what you are looking for. Some examples are
▶ find.byType
▶ find.byKey
▶ find.text
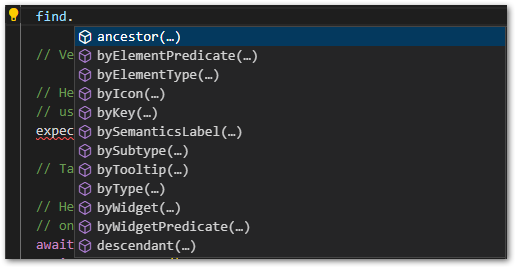
Verification
For verification, you use the expect library like in regular unit tests. A typical expect statement can look like this:
expect(find.byText(”test”), findsOneWidget);
Look for one or more specific widgets and assert it.
Putting it all together
The general workflow in widget tests is always the same. First, you use a Finder to identify a widget you want to interact with. Then, you pass it to the WidgetTester that performs an action and triggers animation and rendering. Finally, you verify the outcome with the Expect class.
The test example is shown below. The entire app source code will be linked at the end.
Conclusion
With this guide, you should be able to write widget tests that verify your app’s correct behavior.
Source code
You can find the source code on GitHub.
Get the Flutter Test Guide for free!
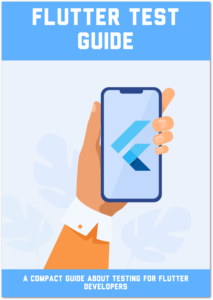
An ebook about unit and widget testing in Flutter applications. Boost your testing skills now with this guide!
Related articles
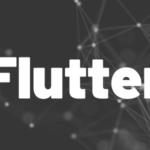
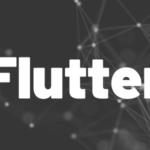