Introduction
Learn how to create a nice mouse hover effect in a Flutter app to make it more appealing on the desktop and web platform.
Since Flutter is gaining more and more traction in the desktop and web world, I wanted to share this guide about mouse hover effects and animations. You will learn how to create a nice mouse hover effect in a Flutter app that will make a widget bigger on hover and return to its original size on exit.
As hovering requires a pointing device like a mouse, it will only work on platforms that support this kind of input behaviour. It’s not suited for mobile apps optimized for touch gestures!
Here is the full example code:
First, we add the SingleTickerProviderStateMixin to our state class. The SingleTickerProviderStateMixin
is a mixin in Flutter that provides a single Ticker
. A Ticker
is essentially a timer that “ticks” every frame, allowing you to run animations or other periodic tasks in your Flutter app. If you have multiple animations running at the same time, use TickerProviderStateMixin instead.
class _State extends State<HoverTest> with SingleTickerProviderStateMixin
initState
In the initState
method, we initialize the AnimationController and the Animation. The controller needs a Duration
and the vsync
property that we can simply assign this
since we include the mixin I previously mentioned. The animation is a Tween
of type double
, has a begin
and an end
property, and needs the animation controller passed as an argument when animate()
is called.
late AnimationController controller;
late Animation<double> myAnimation;
@override
void initState() {
super.initState();
controller = AnimationController(
vsync: this, duration: const Duration(milliseconds: 300));
myAnimation = Tween<double>(begin: 72.0, end: 96.0).animate(controller);
}
dispose
The dispose
method takes care of cleaning up the animation controller. Don’t forget this or your app will have memory leaks.
@override
void dispose() {
controller.dispose();
super.dispose();
}
In the build
method, we have an AnimatedBuilder that builds the widget to animate. It’s an Icon
widget in our case. Since we want to animate the size of the icon, we set the size
property to the current animation value. We declared the animation property as Tween<double>
because the size property of the Icon
widget is of type double
.
build method
To start the animation on mouse hover, we wrap the widget in a MouseRegion
and handle the onEnter
and onExit
methods. When the cursor enters the widget, we want it to animate from the small size (our begin
value) to the big size (our end
value) and when the cursor leaves the widget, the opposite should happen. If you want, you can also change the cursor with the cursor
property of the MouseRegion
widget.
@override
Widget build(BuildContext context) {
return Center(
child: SizedBox(
width: 100,
height: 100,
child: MouseRegion(
cursor: SystemMouseCursors.click,
onEnter: (_) => _hover(true),
onExit: (_) => _hover(false),
child: AnimatedBuilder(
animation: myAnimation,
builder: (context, child) =>
Icon(Icons.photo, size: myAnimation.value)))));
}
void _hover(bool isHovered) {
if (isHovered) {
controller.forward();
} else {
controller.reverse();
}
}
And here is the result:
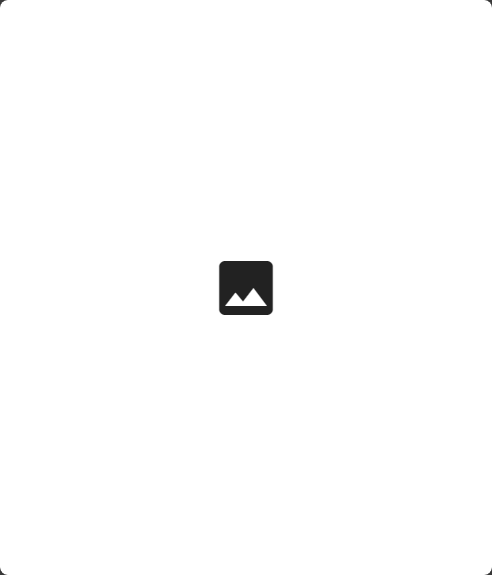
Conclusion
In this article, you learned how to create a nice mouse hover effect in a Flutter app that makes a widget bigger on hover and smaller on exit. A few lines of code is everything you need to achieve this amazing result.
Related articles
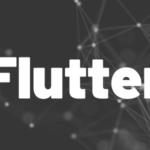
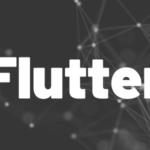