Introduction
I introduced an AbsorbPointer widget which broke my app but the tests to prevent exactly this didn’t fail. Here is why AbsorbPointers are dangerous in Flutter widget tests.
Once again, I stumbled across a weird issue with Flutter widget tests. They are not as reliable as you might think. This time I found a problem with a combination of AbsorbPointer
and TextField
.
Here is a pretty simple example:
@override
Widget build(BuildContext context) {
return AbsorbPointer(absorbing: true, child: TextField());
}
We have a TextField
widget wrapped inside an AbsorbPointer
widget. The purpose of the AbsorbPointer
is to prevent the TextField
from getting focused. The keyboard doesn’t open and you cannot enter text.
When you launch this on any platform (mobile, web desktop), you’ll notice that you cannot focus the input field. Works as expected!
But now, let’s write a simple widget test to ensure the correct functionality. Again some code:
testWidgets('Absorb pointer test', (WidgetTester tester) async {
await tester.pumpWidget(const MyApp());
await tester.enterText(find.byType(TextField), "test");
await tester.pump();
expect(find.text("test"), findsNothing);
});
The test tries to enter text in the TextField
widget and verifies that it cannot find the entered text.
❌ But this test fails!
The expect
line fails because the test could find the text “test”.
If you try the same with another widget like an ElevatedButton
, you don’t get this weird behavior when tapping it. Instead, the error says that your widget cannot receive pointer events which is correct.
Here is the explanation from ChatGPT:
Widget tests simulate interactions at a higher level and don’t rely on actual pointer events or the platform’s keyboard. In your real app, the AbsorbPointer truly blocks the pointer events from reaching the TextField (preventing focus and the keyboard from opening), but in the test environment, the simulated tap doesn’t go through the normal hit testing. Instead, it directly triggers the TextField’s focus logic, so your tests can still enter data.
In short: the AbsorbPointer stops real user taps, but the test framework’s simulated gestures bypass that, allowing your test to work as expected.
I’d be cautious when using AbsorbPointer
widgets in combination with widget tests. AIs seem happy to promote them when asked how to disable tap events on widgets. And since I couldn’t find any bug reports about this, they cannot know the issues.
Conclusion
In this article, you saw why AbsorbPointers are dangerous in Flutter widget tests. It can happen that you change your code and break your app while your tests remain silent and keep working. This happened to me because I blindly trusted AI and my tests.
Related articles
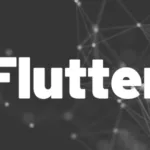
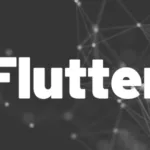