Introduction
In this article, we are having a look at location services and how we can get the current position of the device in our Flutter application.
Location information can be used in various app scenarios. Maybe you want to show points of interest to the users or guide them towards a specific area. Either way, you will need access to the current location of the device. I’ll show you how to accomplish this task.
Setup
The first step is the installation of a package. There are many packages available at pub.dev, but I’ll demonstrate the steps with the most popular one: location. The setup process should be quite similar for all packages, the code however might be slightly different.
Not sure how to install a package? Check the following article for instructions.
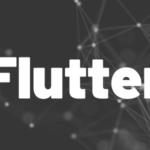
We also need to add capabilities to our app, so that the location request can be granted by the operating system and the user.
For Android, go to android/app/src/main/AndroidManifest.xml
and add the capability
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
or
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
to the file. The first one is more accurate but also requires more battery power. The entry should inside the <manifest>
tag but not inside the <application>
tag.
For iOS, go to ios/Runner/Info.plist
and add the following code inside the <dict>
node.
<key>NSLocationWhenInUseUsageDescription</key>
<true/>
Code
Here is a simple code example that initializes the plugin. You can call it for example in your initState()
method of your main app state.
The following steps are performed:
- Check if device location services are enabled
- Check if usage permission for location services was granted
- Output the current position to the debug console
❗
Warning
If location services are disabled, you will be asked to activate them.
❗
Warning
If usage permission has not been granted yet, you will be asked on Android. iOS will always ask on app launches. If you deny the usage, you won’t be prompted again. To change the setting, you’ll have to go to your phone settings and enable the permission for this app.
To get continouos information about position changes, you can add a listener.
location.onLocationChanged.listen((LocationData loc) {
print("${loc.latitude} ${loc.longitude}");
});
Conclusion
In this article, I showed you how to set up and work with location data. The configuration can be a bit tricky at first, but the possibilities of location data are endless.
Related articles
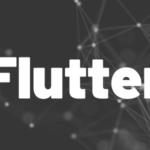
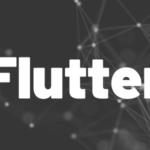