Introduction
Learn how to prevent RichText
styling issues in Flutter apps and consider using the alternative Text.rich
. This small tip will save you time!
Flutter provides developers with a versatile set of widgets for creating rich and engaging user interfaces. Two commonly used widgets for displaying styled text are RichText
and Text.rich
. While both widgets offer text styling capabilities, it’s essential to understand their differences, especially the fact that RichText
does not use the default text theme. Learn how to prevent RichText styling issues in Flutter to not get headaches like did.
RichText Widget
The RichText
widget in Flutter is designed to display a paragraph of mixed-style text. It allows you to apply different text styles to different parts of the text within a single widget. This makes it an excellent choice for situations where you need to format text in various ways within the same text block.
One important distinction to note is that RichTex
t
does not inherit the default text theme of your app. This means that if you want to apply a specific text style to your RichText
, you need to explicitly define it for each TextSpan
within the widget. While this may require a bit more work compared to using Text
with the default text theme, it grants you full control over the styling of individual text spans.
Here’s a simple example of how to use RichText
:
RichText(
text: TextSpan(
text: 'This is an example of ',
style: TextStyle(color: Colors.black),
children: <TextSpan>[
TextSpan(
text: 'richly styled text',
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.blue,
),
),
TextSpan(
text: ' in Flutter.',
style: TextStyle(
fontStyle: FontStyle.italic,
),
),
],
),
)
In this example, we have three different text spans with varying styles, all displayed within the same RichText
widget.
Text.rich Widget
The Text.rich
widget is an alternative to RichText
that simplifies text styling by making use of the default text theme. Unlike RichText
, Text.rich
inherits the text style of your app’s theme, making it a convenient choice for maintaining consistency in your app’s design.
Here’s how you can use Text.rich
:
Text.rich(
TextSpan(
text: 'This is an example of richly styled text in Flutter.',
children: <TextSpan>[
TextSpan(
text: ' You can apply different styles easily.',
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.blue,
),
),
],
),
)
In this example, the Text.rich
widget automatically uses the default text theme’s style, but you can still override it for specific text spans as needed.
Want More Flutter Content?

Join my bi-weekly newsletter that delivers small Flutter portions right in your inbox. A title, an abstract, a link, and you decide if you want to dive in!
Comparison
Here is the output of the code examples from above.

As you can see, they use different fonts. Since we didn’t specify the fonts in the widget, I would assume that the default text theme font is applied. But this is not the case for RichText
. My advice is to only use Text.rich
to prevent this problem.
I didn’t spot other properties than the font so far, but maybe there are more to be aware of. With Text.rich
you should be on the safer side.
Conclusion
In this article, you learned how to prevent RichText styling issues in Flutter to not get headaches like I did.
Related articles
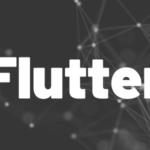
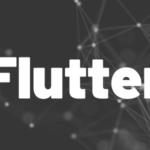