Introduction
The Firebase Analytics Debug View is a handy tool to check what events your app sends in real time. However, it requires a bit of setup to work from a Flutter web app. Here is how to debug Firebase Analytics events from a Flutter web app.
Setup
The initial step is to connect your Flutter app with your Firebase project. Follow my guide in case you haven’t done this yet.
To work with Firebase Analytics, you then need to install the firebase_analytics and firebase_analytics_web (only for Flutter web apps) packages.
In your code, get a reference to Firebase Analytics like this:
final FirebaseAnalytics _analytics = FirebaseAnalytics.instance;
After that, you can log events. Here are some examples:
// user opens the app
_analytics.logAppOpen();
// user clicks on a promotion element
_analytics.logSelectPromotion(creativeName: productName);
// user activates a product upgrade
_analytics.logEvent(name: "product_activation", parameters: {"license_key": licenseKey});
Firebase Analytics offers predefined operations but when you have custom events, the generic logEvent() method is your friend.
Using the Debug View
The Firebase Analytics Debug View is part of the Firebase Console. In your project go to Analytics -> Debug View to open it.
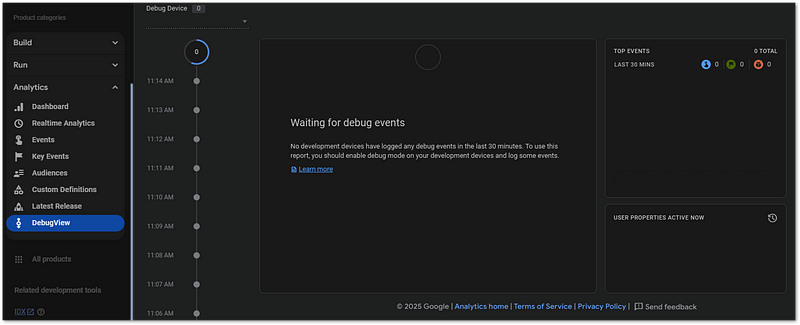
When you run your app directly from VS Code and trigger events, nothing will show up in the debug view. To make it work, you need to install the Google Analytics Debugger as a browser extension.
Launch your Flutter app on a web server with the command
flutter run -d web-server
The command shows an url to access the instance. Open it with your browser that has the Google Analytics Debugger installed and enabled.
💡 Tip
Why do you need to launch a web server?
When VS Code opens a browser window with your web app, it uses
a temporary profile which has no extensions installed. That’s
why the events are not reported to the Firebase Analytics Debug View.
Trigger some events to test it. It might take up to 15 seconds for the events to appear. Eventually, you should see something like this:
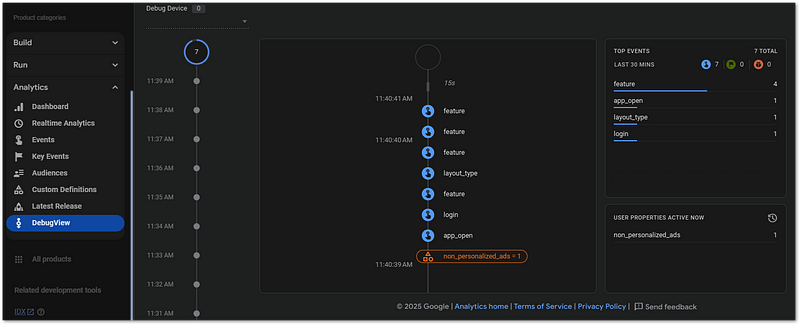
And this is how to debug Firebase Analytics events from a Flutter web app.
Conclusion
In this article I showed you how to debug Firebase Analytics events from a Flutter web app. It’s not as simple as when testing mobile apps but once you know how it works, it’s not a big deal anymore.
Related articles
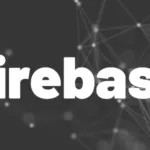
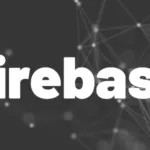