Introduction
Reduce database load and add offline capabilities to your apps. Here is how to enable Firestore cache in Flutter web apps. If you target Android or iOS, caching is enabled by default!
In case you didn’t know yet: Firebase Firestore can be used offline by enabling an internal cache. Your read and write operations work normally in offline mode and will synced with the database once the device is online again. This feature is enabled on Android and iOS by default. But for web apps you need to enable it yourself. Here is how to enable Firestore cache in Flutter web apps.
Enable persistence
Web apps don’t have persistence enabled by default because the browser might not support it. Currently, you are fine with Chrome, Safari, Firefox, and Edge. To enable persistance, use the following code:
await FirebaseFirestore.instance
.enablePersistence(const PersistenceSettings(synchronizeTabs: true));
This must be the first call to Firestore after your app launches. Otherwise, it will fail with the following error message:

With the synchronizeTabs
parameter you can specify how your app should handle multiple tabs. If this value is false and a user opens your app in more than one tab, this call will fail after the first time. You could then show a message to only use your app in one tab at a time for example.
To set the cache size, use the following code:
FirebaseFirestore.instance.settings =
const Settings(cacheSizeBytes: Settings.CACHE_SIZE_UNLIMITED);
However, you should be fine with the default value in general. When the cache is full, the oldest unused documents get cleaned up first.
Fix hot reload/hot restart error
Flutter developers love hot reload and hot restart to quickly see the changes they made to their apps. But when you enable persistence in a Flutter web app, you’ll be greeted with the already mentioned error message:
Firestore has already been started and persistence can no longer be enabled. You can only enable persistence before calling any other methods on a Firestore object.
Hot reload/hot restart don’t reset Firestore. That’s why you get the error. Closing and restarting the entire app works but it consumes a lot of time. On my system a hot reload is about 500ms, a hot restart about 2-3 seconds, and full restart takes up to 30 seconds.
Since there is no built-in solution in Firebase, I came up with this one:
try {
await FirebaseFirestore.instance
.enablePersistence(const PersistenceSettings(synchronizeTabs: true));
} catch (_) {
}
I simply wrap it in a try catch block and swallow the exception. This approach also works for hot reloads/hot restarts and I don’t need to wait for my app to completly shut down and boot up again. I don’t care if it’s perfect or not because it’s only relevant when debugging apps and I needed a way to make hot reload/hot restart work again.
Flutter ❤️ Firebase
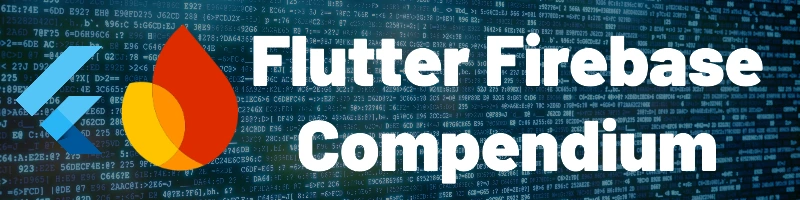
Get started with Firebase and learn how to use it in your Flutter apps. My detailed ebook delivers you everything you need to know! Flutter and Firebase are a perfect match!
Conclusion
In this article, I showed you how to enable Firestore cache in Flutter web apps. It’s a mighty feature that can save you money and add offline capabilities with just one line of code.
Related articles
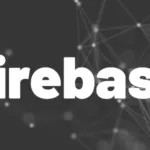
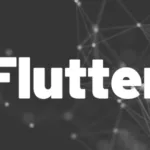