Introduction
With Firebase Extensions you can build a simple text translation app that does most of the work for you.
There are many ways to build a translation app. In this article, I am going to show you how to use Firebase for that task. A Flutter app will transmit the text to be translated to Firebase and display the final result. We will use Firebase Cloud Firestore and a Firebase Extension called Translate Text for this purpose.
🔔 Hint
Be aware that you might run into some costs. An installed extension costs around 1 cent per month and it normally uses other services in the background which can increase these costs. You will also need to activate the Blaze plan.
Firebase setup
First, we need to install the required extension. Go to Build → Extensions.
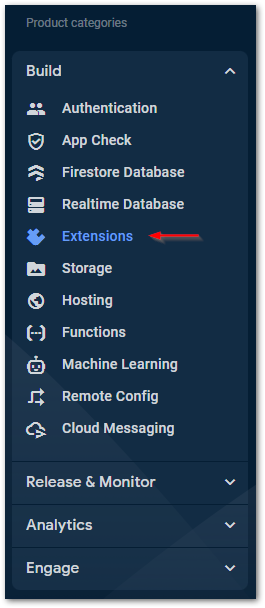
Afterward, find the extension Translate Text and install it.
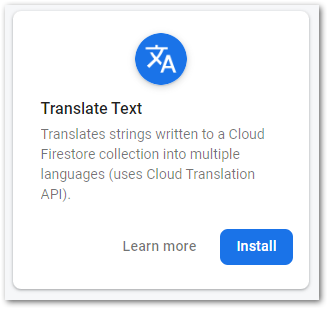
Follow the wizard. During the process, you might encounter hints for additional services that need to be enabled for the extension to work.
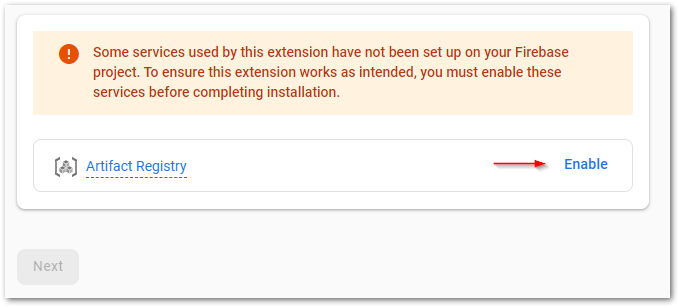
Configure the extension to your needs or use the default values. A guide on how to select the best location can be found here.
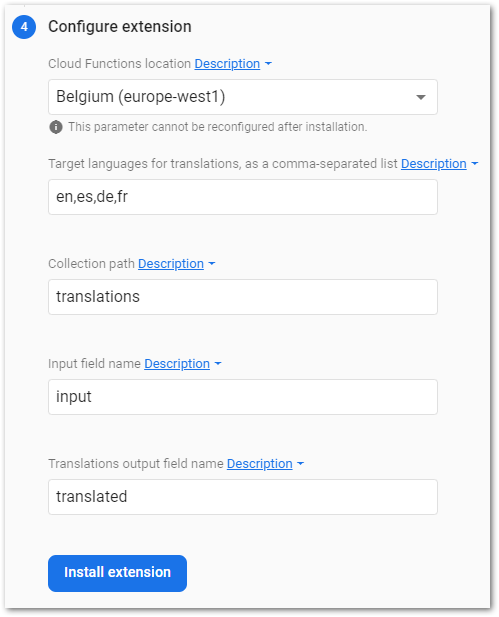
The process will take some minutes to complete.

You can see the details of the extension in the Extensions dashboard in Firebase. There is also an explanation of how to set up Cloud Firestore.
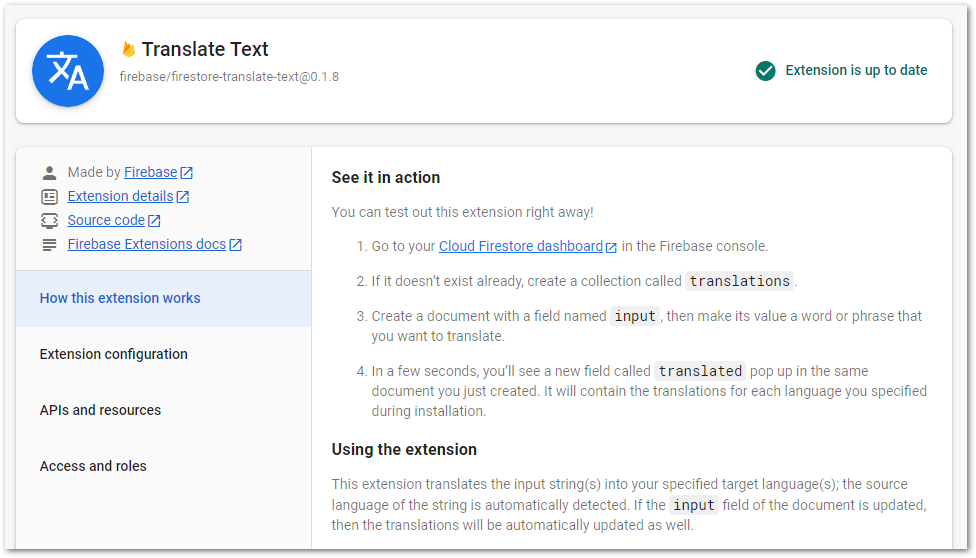
Now, we switch to the Cloud Firestore database. According to the instructions we create a new collection called translations and a document with a field called input. The value of the input field will be translated to the configured languages and stored in the translated field.
🔔 Hint
What happens behind the scenes? When the field value changes, a cloud function is triggered (you can see it in the Cloud Functions Dashboard of Firebase). It uses the Cloud Translation API to translate the text and writes the result back to the Cloud Firestore document.
The Firebase setup is completed. Let’s write a simple Flutter app to make use of our workflow.
Want More Flutter Content?

Join my bi-weekly newsletter that delivers small Flutter portions right in your inbox. A title, an abstract, a link, and you decide if you want to dive in!
App setup
We need to create an app that writes text to Cloud Firestore and listens to changes which it will then display to the user.
Step 1: Create a Flutter project.
Use the command flutter create my_sample_project
or your IDE of choice for it.
Step 2: Link it with your Firebase project.
Run flutterfire configure
from the project root folder and follow the wizard.
Step 3: Install Firebase packages.
We need the Core package and since we want to use Cloud Firestore, we need that package, too.
Step 4: Add Firebase initialization code.
Add
await Firebase.initializeApp(options: DefaultFirebaseOptions.currentPlatform);
to main function and make it asynchronous.
Step 5: Build a simple UI.
I’ll go with one TextField widget, one ElevatedButton widget, and 4 Text widgets inside of a StreamBuilder for the translations.
Step 6: Connect UI to Cloud Firestore.
The button sends the text to Firestore and the UI listens for changes. Here’s some code:
And this is what the final example can look like:
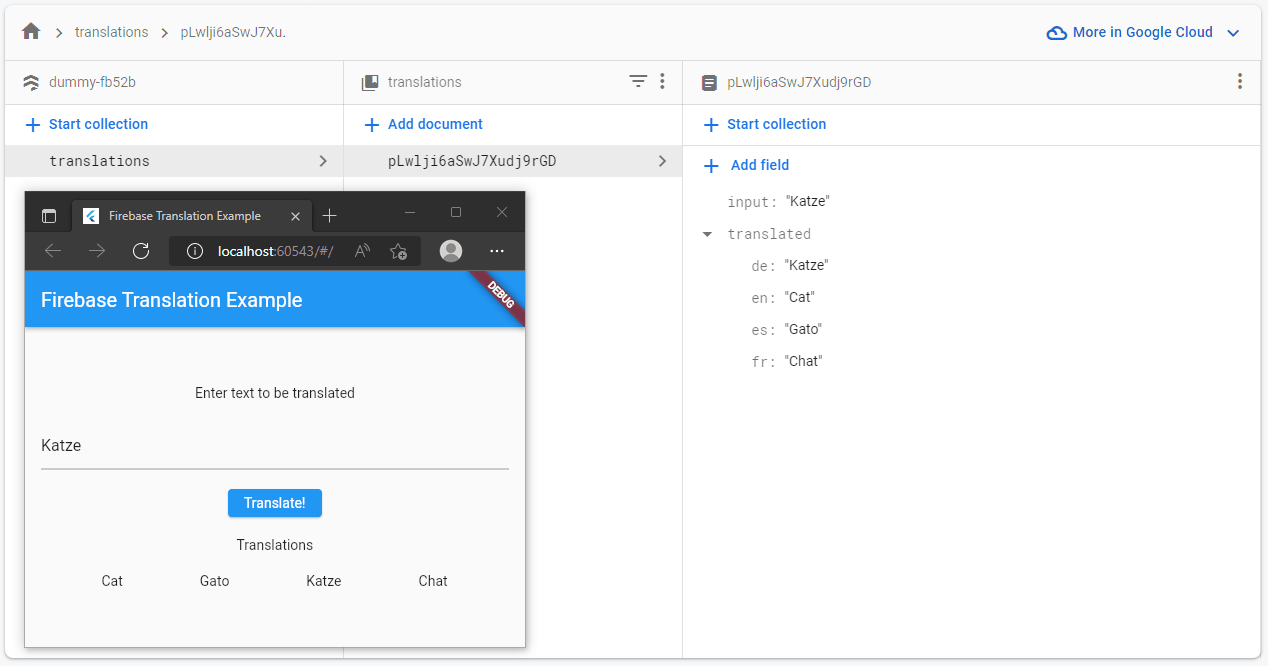
It will take you an hour at most to get to this result. A translation feature that can be integrated in any app.
How much is it?
As mentioned earlier, this workflow is not for free. You will be charged for the following reasons:
▶ Firebase resources of the extension (around 1 cent per month)
▶ Cloud Firestore
▶ Cloud Functions
▶ Cloud Translation API
▶ Additional Firebase and Google Cloud Platform services
Some of these services have free quotas (Cloud Firestore for example) but not all of them. The final price is always connected to the number of users your app will have. Monitoring your expenses is highly recommended.
If you are just experimenting with Firebase, you won’t get charged more than some cents per month.
Level up your Firebase skills!
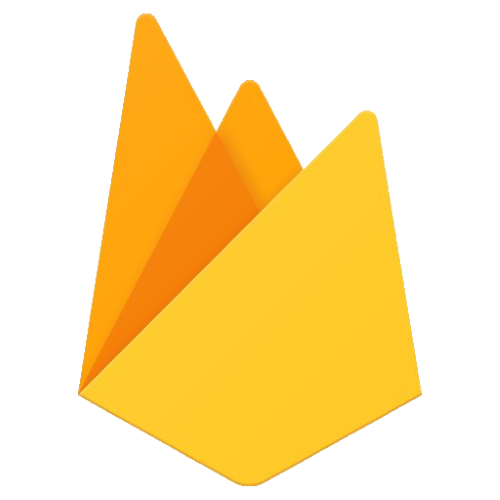
Check out my free email course on Firebase fundamentals, and grab your copy of my Firebase ebooks. Start building amazing apps with Firebase today!
Additional resources
Here are some additional resources about Firebase in case you want to dive deeper into the topic.
Firebase Cloud Functions
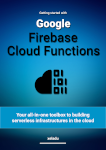
Your all-in-one toolbox to building serverless infrastructures in the cloud. Write once and scale to infinity!
Firebase Cloud Storage
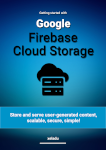
Upload and download user-generated content like on a file system. Firebase Cloud Storage makes file handling simple!
Firebase Remote Config
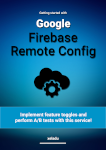
Real-time feature toggles or A/B testing are typical use cases of Firebase Remote Config. Learn how to implement them now!
Firebase Console
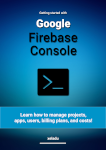
Learn how to manage projects, apps, users, billing plans, and costs with step-by-step guides in the Firebase Console.
Firebase Cloud Firestore
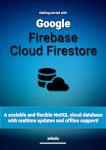
Learn about Firebase Firestore and write mobile apps with the power of a modern and fast NoSQL database.
Firebase Authentication
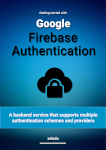
Implement email/password authentication or use social providers like Google, Microsoft, and Facebook for your apps!
Firebase Hosting
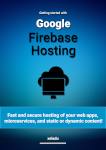
Host your web apps, microservices, dynamic, and static content with this powerful yet simple solution from Firebase!
Conclusion
This is a small example of what you can build with Firebase and its features. The source code of the Flutter app is available on GitHub.
Related articles
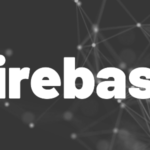
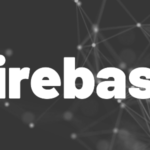