Introduction
In this article, I’ll show you how to store Flutter app data on your device with the plugin shared_preferences. It’s the default solution when you want to store some data!
Shared Preferences is a useful tool for persisting small amounts of data, such as user settings. It’s a key-value store similar to a Dictionary
in Dart. The API is easy to learn, with getter and setter methods for various types like Int
, Double
, Bool
, String
, and StringList
. Let’s learn how to store Flutter app data on your device with it!
It’s available for all major platforms like Android, iOS, macOS, Windows, Linux, and web. Under the hood, it uses the native SDKs provided by the operating system.
Reading and writing primitive data
Shared Preferences stores the data on the local hard drive. When you relaunch your app, you can load the data from disk and continue working as before.
Here is a code example showing how to read and write data with the Shared Preferences package:
Working with complex objects
To store complex data objects, we use the following approach:
- Implement your custom class
- Add a
fromJson
factory constructor that constructs an object from aMap<String, dynamic>
- Add to
toJson
method that returns aMap<String, dynamic>
from your object - Save it with
await prefs.setString("key", jsonEncode(myObject.toJson()))
- Load it with
CustomClass.fromJson(jsonDecode(prefs.getString(”key”)))
Here is a short example of such a class:
With this approach, you can store any complex object with the Shared Preferences plugin.
Testing
When you have tests that rely on Shared Preferences, you can use a mock implementation. The following code shows how to set values for testing:
final Map<String, Object> values = <String, Object>{'counter': 1};
SharedPreferences.setMockInitialValues(values);
In this test mode, Shared Preferences will not store any data on persistent storage. All operations only work in memory.
Conclusion
In this article, you learned how to store Flutter app data on your device with the plugin shared_preferences. It works well with primitive and complex data types and has great testing support.
An In-Depth Firebase Guide For Flutter Developers!
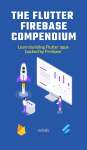
This compendium is a comprehensive guide with many step-by-step guides and code examples. Learn the essentials of Firebase and enhance your apps with cloud services in no time!
Related articles
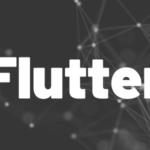
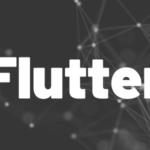