Let’s have a look at how to use Flutter image picker and Firebase storage to save your images in the cloud!
Saving images in the cloud is a convenient way to ensure that it is available on any device. In this article, I want to show you how to use Flutter image picker and Firebase storage to do exactly that. We will pick a file (or take a picture), upload it to Firebase Storage, and display it in our app. Let’s get started!
Image picker
Our app needs some packages to make it as easy as possible for us. The first one is image_picker. It offers a dialog to select a file from your gallery or take a picture if your device has a camera. This works on all major platforms. Here is how you use it:
final image = await ImagePicker().pickImage(source: ImageSource.gallery);
if (image != null) {
final imageFile = await image.readAsBytes();
...
}
You can also use ImageSource.camera
to trigger the camera dialog. After you have taken a picture, it will be returned by the pickImage()
method. Usually, you would trigger this when the user clicks a button or something like that.
We have our image. Let’s upload it to Firebase and display it in our app!
Firebase
We need the packages firebase_core and firebase_storage. I assume you are familiar with creating a Firebase project and configuring Firebase Storage. If not, check out this introduction article.
Here is how you can upload your file to Firebase Storage:
final image = await ImagePicker().pickImage(source: ImageSource.gallery);
if (image != null) {
final imageFile = await image.readAsBytes();
final ref = FirebaseStorage.instance.ref();
final child = ref.child("images/${image.name}");
final uploadTask = child.putData(imageFile);
...
}
This will upload the selected image to a subfolder images
in Firebase Storage.
The last thing is to display the image.
Display image
Throughout our journey on how to use Flutter image picker and Firebase Storage, we have successfully selected an image using a dialog and uploaded it to Firebase. Now, we aim to display this image in a Flutter app.
We use the uploadTask
from before and wait until it tells us that the upload has completed successfully.
final image = await ImagePicker().pickImage(source: ImageSource.gallery);
if (image != null) {
final imageFile = await image.readAsBytes();
final ref = FirebaseStorage.instance.ref();
final child = ref.child("images/${image.name}");
final uploadTask = child.putData(imageFile);
uploadTask.snapshotEvents.listen((event) async {
if (event.state == TaskState.success) {
_imagePath = await event.ref.getDownloadURL();
setState(() {});
} else if (event.state == TaskState.error) {
// handle error case
}
});
}
When completed, we retrieve the download url and store it in a field.
In our build
method, we have an Image
that waits for this download url. While the url is null or empty, we just display nothing. It could look like this:
String _imagePath = "";
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(child: _imagePath.isNotEmpty
? Image.network(_imagePath)
: const SizedBox()));
}
And this is it! We selected an image with a file picker, uploaded it to Firebase Storage, and displayed it in our Flutter app.
With this basic example code, you can enhance your app and make it cloud-ready.
Firebase Cloud Storage
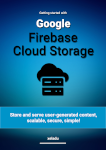
Upload and download user-generated content like on a file system. Firebase Cloud Storage makes file handling simple!
Conclusion
In this article, you learned how to use Flutter image picker and Firebase Storage to save your images in the cloud. With the provided code examples, you should be able to get started quickly and enhance your app the way you need it to be.
Related articles
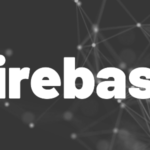
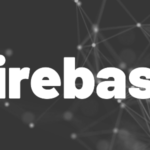